An Introduction to Real-World Programming with Java: A Comprehensive Guide for Beginners

Java is one of the most popular programming languages for real-world applications. It's a versatile language that can be used to develop a wide variety of software applications, from small desktop programs to large-scale enterprise systems. If you're interested in learning how to program in Java, this article will provide you with a comprehensive to the language and its real-world applications.
4.3 out of 5
Language | : | English |
File size | : | 15481 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 848 pages |
X-Ray for textbooks | : | Enabled |
What is Java?
Java is an object-oriented programming language that was developed by Sun Microsystems in the early 1990s. It's a high-level language, which means that it's easy to read and write, and it's also platform-independent, which means that it can be run on any operating system that has a Java Virtual Machine (JVM) installed.
Why Java?
There are many reasons why Java is a good choice for real-world programming. First, it's a very versatile language that can be used to develop a wide variety of applications. Second, it's a high-level language that's easy to read and write. Third, it's platform-independent, which means that it can be run on any operating system. Fourth, it's a very powerful language that can be used to develop complex applications.
Getting Started with Java
If you're interested in learning how to program in Java, the first step is to install the Java Development Kit (JDK). The JDK is a software package that includes the Java compiler, the Java Virtual Machine, and other tools that you need to develop Java programs. Once you have the JDK installed, you can start writing Java programs using any text editor. However, it's recommended to use a Java integrated development environment (IDE),such as Eclipse or IntelliJ IDEA, which can provide you with a number of features to make it easier to develop Java programs.
Hello World!
The following is a simple Java program that prints the message "Hello, world!" to the console:
java public class HelloWorld { public static void main(String[] args){System.out.println("Hello, world!"); }}
To compile and run this program, you can use the following commands:
javac HelloWorld.java java HelloWorld
This will compile the HelloWorld.java file into a HelloWorld.class file and then run the HelloWorld class.
Data Types
Java has a variety of data types that can be used to store different types of data. The most common data types are:
- byte: 8-bit signed integer
- short: 16-bit signed integer
- int: 32-bit signed integer
- long: 64-bit signed integer
- float: 32-bit floating-point number
- double: 64-bit floating-point number
- boolean: true or false
- char: 16-bit Unicode character
- String: a sequence of characters
Variables
Variables are used to store data in Java. You can declare a variable by using the following syntax:
java
For example, the following code declares a variable named `age` of type `int`:
java int age;
You can assign a value to a variable using the assignment operator (=).
java age = 25;
Operators
Java has a variety of operators that can be used to perform different operations on data.
There are many different types of operators in Java, but some of the most common are:
- Arithmetic operators: +, -, *, /, %
- Assignment operators: =, +=, -=, *=, /=
- Comparison operators: ==, !=, , =
- Logical operators: &&, ||, !
Control Flow
Control flow statements are used to control the flow of execution in a Java program.
There are many different types of control flow statements, but some of the most common are:
- if statements
- switch statements
- for loops
- while loops
- do-while loops
Methods
Methods are used to perform specific tasks in Java.
You can define a method by using the following syntax:
java
For example, the following code defines a method named `greet` that takes a name as a parameter and prints a greeting to the console:
java public static void greet(String name){System.out.println("Hello, " + name + "!"); }
You can call a method by using the following syntax:
java
For example, the following code calls the `greet` method with the argument "John":
java greet("John");
Classes
Classes are used to define objects in Java.
You can define a class by using the following syntax:
java public class
For example, the following code defines a class named `Person`:
java public class Person { private String name; private int age;
public Person(String name, int age){this.name = name; this.age = age; }
public String getName(){return name; }
public int getAge(){return age; }}
You can create an object by using the `new` keyword:
java Person person = new Person("John", 25);
Inheritance
Inheritance is a mechanism that allows you to create new classes from existing classes, reusing the code and functionality of the existing classes.
To define a new class that inherits from an existing class, you can use the following syntax:
java public class
For example, the following code defines a class named `Employee` that inherits from the `Person` class:
java public class Employee extends Person { private int salary;
public Employee(String name, int age, int salary){super(name, age); this.salary = salary; }
public int getSalary(){return salary; }}
Polymorphism
Polymorphism is a mechanism that allows you to write code that can work with different types
4.3 out of 5
Language | : | English |
File size | : | 15481 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 848 pages |
X-Ray for textbooks | : | Enabled |
Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
Fiction
Non Fiction
Romance
Mystery
Thriller
SciFi
Fantasy
Horror
Biography
Selfhelp
Business
History
Classics
Poetry
Childrens
Young Adult
Educational
Cooking
Travel
Lifestyle
Spirituality
Health
Fitness
Technology
Science
Arts
Crafts
DIY
Gardening
Petcare
J Bruce Brackenridge
Reelav Patel
Catherine Shainberg
Samantha Fitts
Spencer Wells
Dr Craig Malkin
Mike High
Hunbatz Men
Chris Mooney
Grey Owl
Yuval Noah Harari
Chiara Sparks
Isabel Fonseca
Julie L Spencer
Joshua Hammer
Ryan A Pedigo
Eric Engle
Christopher Taylor Ma Lmft
Nicholas Sparks
Mark Twain
Benita Bensch
Marco Grandis
Charles River Editors
Sampson Davis
Editors Of Southern Living Magazine
Barry Rhodes
David Aretha
Scott Mactavish
Martin Dugard
Marie Rutkoski
Helen Kara
V B Alekseev
Gerald Beaudry
Barzin Pakandam
Rob Hutchings
W Hamilton Gibson
Janna Levin
John G Robertson
Kenton Kroker
Kathleen Masters
Laura Ingalls Wilder
Thomas Bulfinch
Charles Soule
R L Medina
Kate Darling
Jay Abramson
Randy Baker
Kyle Butler
Craig Martin
Gordon H Chang
Carlo Collodi
Tara Brach
Martin Sternstein
Blair Braverman
Sean Mcindoe
Jen Houcek
Peter Wacht
Bryan Peterson
Vanessa Ogden Moss
James Syhabout
John C Norcross
Mark H Newman
Nadine Hays Pisani
Jonathan Bergmann
Sharon Dukett
Tom Miller
Daniel J Barrett
Tim Marshall
Christine Kenneally
Jean Illsley Clarke
Rick Joyner
Farley Mowat
Richard Chun
Jesse Liberty
Conor Nolan
Yuki Mano
Hill Gates
Charlie Shamp
Becca Anderson
Deanna Roy
Achille Rubini
Gordon Witteveen
Barry Friedman
Jenn Mcallister
Susan Dennard
Jean Smith
Jayanti Tambe
Dave Rearwin
Jeffrey Jensen Arnett
Ian Leslie
Mackenzi Lee
Erica Schultz
Cary J Griffith
Charlotte Browne
Cara Koscinski
Siddhartha Rao
Barbara Gastel
Dr Faith G Harper
Lock Gareth
Basudeb Bhatta
Kit Yates
Chris Cage
Michelle Rigler
Chase Hill
Pavla Kesslerova
Maha Alkurdi
Thomas French
David Thomas
Percy Boomer
Dr Michael P Masters
Chris Bennett
Matt Taddy
Catherine M Cameron
Edith Grossman
Sara Shepard
Joseph Epes Brown
Bb
Mark Young
Geoffrey Finch
Charlotte Booth
Jessica Smartt
Craig Lambert
Steven Hawthorne
Brad Burns
Barry J Kemp
Kate Williams
Lily Collins
Jack Newman
Bagele Chilisa
Rob Rains
Edwin R Sherman
Proper Education Group
Margaret Owen
Ascencia
Macauley Lord
Arny Alberts
Marshall Jon Fisher
Robert Hogan
Lawrence Baldassaro
Simon Spurrier
Eric Franklin
Mark Kurlansky
Jake Anderson
Colleen Alexander Roberts
Sergei Urban
Kindle Edition
Jim Wharton
Lynn Rosen
Christopher L Heuertz
Joshua G Shifrin
Bill Milliken
Ralph Galeano
Rhonda Belle
Jenny Chandler
David A Bogart
Bridget Flynn Walker Phd
Jessica F Shumway
C R Hallpike
John Henry Phillips
Alastair Hannay
Ronald Wheeler
Kara Goucher
W Scott Elliot
Burt L Standish
Catherine J Allen
Nicole Martin
Jude Currivan
Jeff Belanger
David Abram
Jaymin Eve
Harvey Wittenberg
Stephen J Bavolek
Breanna Hayse
Stefan Ecks
Joseph Alton M D
Jennifer Estep
Emma Walker
J Michael Veron
Jack Andraka
Susan Nance
Jonathan Kellerman
Linnea Dunne
Erik J Brown
David Beaupre
Daniel S Lobel Phd
Susan Scott
Robin Knox Johnston
Donald Frias
Marc Loy
Monta Z Briant
F William Lawvere
Mick Conefrey
Donald R Gallo
Mike Swedenberg
Ben Povlow
Paul Van Lierop
Steven Bell
Diane Musho Hamilton
Yang Kuang
Eugene V Resnick
Mina Lebitz
Leia Stone
Peter Martin
Elliott Vandruff
Jill Brown
Gabriyell Sarom
Dan Golding
Eric Leiser
Khurshed Batliwala
Robert A Baruch Bush
Denton Salle
John H Mcwhorter
Rachelle Zukerman
Leonard M Adkins
David Burch
Hongyu Guo
Suzanne Leonhard
Bill Mckibben
Melissa Gomes
Jade Barrett
Vanessa Garbin
David Cockburn
Roanne Van Voorst
Diane Yancey
Richard H Immerman
Nancy E Willard
Ray Comfort
Pat Shipman
Robin Mcmillan
Joanne Glenn
Tim Ingold
Barbara Bassot
Jane Hardwicke Collings
Rachel Smith
Scott Westerfeld
Chuck Missler
Steven Rinella
Nichole Carpenter
Edward Lee
Ryan T White
Robin Nixon
Baruch Englard
Lewis Thomas
Sam Harris
John Quick
Mary A Fristad
Monica Hesse
Howard Zinn
Graham R Gibbs
John J Robinson
Leah Cullis
William Byers
Mark Ellyatt
Rachel Dash
Jeff Alt
Kenneth Wilgus Phd
Tori Day
Lynn Mann
Malcolm Hebron
Alexandra Andrews
Stephanie Fritz
Smart Reads
Ben Sedley
Barbara Russell
Oliver T Spedding
Dave Karczynski
Bruce Pascoe
Arnold G Nelson
Clifford Herriot
Peter K Tyson
Daniel M Koretz
Jane Butel
Nick Bollettieri
Patrick Sweeney
Ron Avery
David Jamieson Bolder
Mike Stanton
Daniel T Willingham
Larry Dane Brimner
Joseph Schmuller
Print Replica Kindle Edition
Helen Fisher
Ping Li
Valerie Pollmann R
Barry Burd
Stan Tekiela
Cynthia Levinson
Scott Reed
Roy Porter
Dustin Salomon
Ron Lemaster
Fabien Clavel
Joanna Hunt
Stanislas Dehaene
Janis Keyser
William Wasserman
Sport Hour
John Aldridge
Vincent Bossley
Babu The Panda
Ben Goldacre
Barbara Illowsk
Wayne B Chandler
Titus M Kennedy
Guy P Harrison
Noah Brown
Mark Rashid
Dacher Keltner
Chris Eberhart
Luke Gilkerson
Graham Farmelo
Hollis Lance Liebman
Visual Arts
James Duthie
T H White
Mike Loades
Beebe Bahrami
Emily Chappell
Beau Miles
Barbara Ann Kipfer
Barbara Taylor
Rowan Jacobsen
David Starbuck Smith
Geert Hofstede
Michael Wood
Wanda Priday
Richard Meadows
St Louis Post Dispatch
Jennifer Pharr Davis
Michael Palin
Scott Malthouse
James Koeper
Dan R Lynch
Elizabeth George Speare
Karen Bush
Suzanne Wylde
Richard Weissbourd
Beth Miller
Cindy Post Senning
Autumn Carpenter
Sam Nadler
John Kimantas
Leslie Stager
Eliza Reid
Muhammad Zulqarnain
Crystal Duffy
Bashir Hosseini Jafari
Mike Massie
Glenna Mageau
George Macdonald
Kam Knight
Betty Stone
Julian I Graubart
Master Gamer
Richard J Dewhurst
Jutta Schickore
Molly E Lee
P J Agness
John D Barrow
Jenna Helland
Temple West
James M Collins
John Garrity
Gary Soto
David Taylor
Clayton King
Candice Davie
Mia Scotland
Nick Townsend
Ellen Notbohm
Toby A H Wilkinson
William H Frey
Farzana Nayani
S K Gupta
Barry Johnston
Reprint Edition Kindle Edition
Holger Schutkowski
Barbara Mertz
Gay Robins
Boy Scouts Of America
Barbara Neiman
Mike Commito
Massimo Cossu Nicola Pirina
James Kilgo
Philip Maffetone
Erica B Marcus
Ben Collins
Ken Xiao
Rachel Morgan
Ryan Higa
Nina Manning
Trish Kuffner
Rebecca Rupp
Scarlett Thomas
Thomas Lumley
Robert Ardrey
J C Cervantes
Emma Brockes
Jeremy Sweet
Jamie Margolin
Steve Burrows
Bob Holtzman
Nick Neely
David Klausmeyer
Timothy Pakron
Victor Seow
Anthony Edwards
Cait Stevenson
Elizabeth Lockwood
Mike Allison
Sam Cowen
Debra Kilby
Ellen Lewin
T C Edge
James Duggan
Kara Tippetts
Casey Watson
Richard Harris
Shayla Black
Prince Asare
Charles Buist
Basu Shanker
Ernie Morton
Brandon Royal
Steve Biddulph
Bonnie Tsui
Mark Mayfield
Frederick Douglass Opie
Tami Anastasia
Laura Pavlov
Shannon Reilly
Dustin Hansen
Brian Switek
S E Hinton
Barry Glassner
Leon Mccarron
Buddy Levy
Daniel P Huerta
Linda Welters
Shmuel Peerless
Cheryl Erwin
Chadd Vanzanten
Sam Kean
Mitch Prinstein
Eddie Merrins
Rebecca Solnit
Diane Cardwell
Debbie M Schell
Gary Lincoff
John Lister Kaye
Fred Mitchell
Stephen K Sanderson
Constanze Niedermaier
Bobbie Faulkner
Rob Pope
Otto Scharmer
Beau Bradbury
James Randi
Meghan L Marsac
James C Radcliffe
John Whitman
Nicholas Wolterstorff
Jennifer Traig
Linda Carroll
Craig Romano
Bernard Marr
Mykel Hawke
Lew Freedman
Barbara Rogoff
John Sandford
Pearson Education
Jim Fay
Allan V Horwitz
Max Marchi
Danny Staple
Paul Doiron
Violet Moller
Richard Scott
Julie Buxbaum
Barbara Kennard
Brian Gilbert
Justin Sirois
Barry Rabkin
Melissa Haag
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!
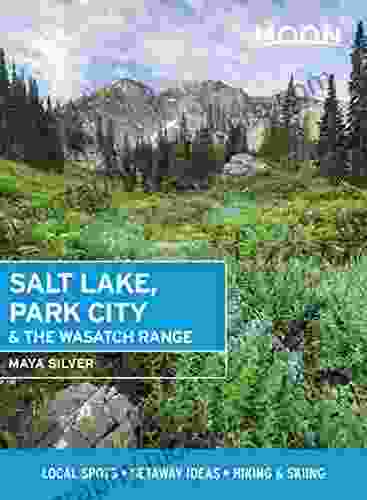

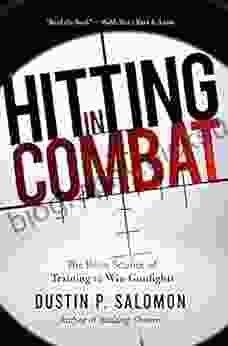

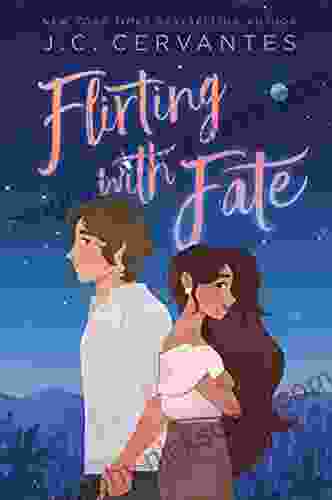

- Cortez ReedFollow ·7.1k
- Stan WardFollow ·3.4k
- Jacques BellFollow ·9.2k
- Eugene ScottFollow ·6.5k
- Cade SimmonsFollow ·16.6k
- Hugh ReedFollow ·12.7k
- Quincy WardFollow ·2k
- Cason CoxFollow ·4.4k
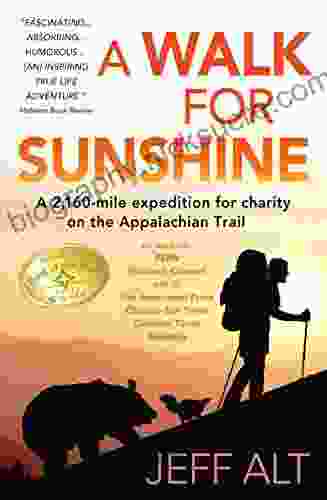

Embark on an Epic 160-Mile Expedition for Charity on the...
Prepare yourself for an...
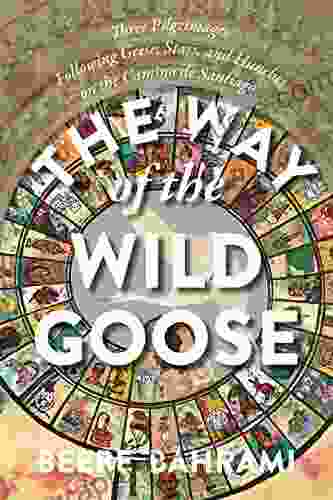

The Way of the Wild Goose: A Journey of Embodied Wisdom...
The Way of the Wild Goose is an ancient...
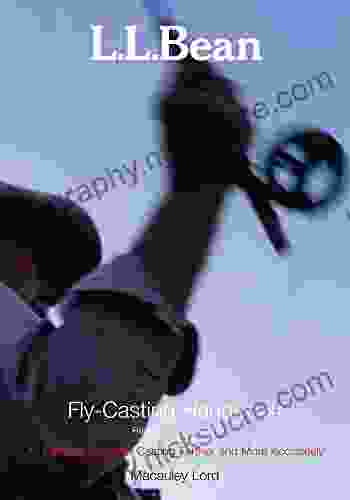

Mastering the Art of Bean Fly Casting: A Comprehensive...
Fly fishing,...
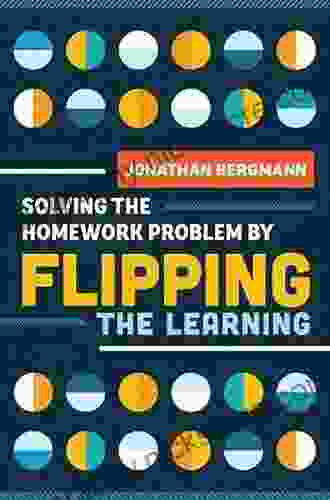

Solving the Homework Problem by Flipping the Learning
What is flipped...
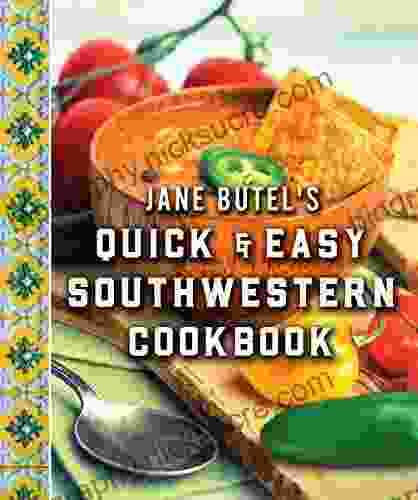

The Jane Butel Library: A Renewed Source of Knowledge and...
The Jane Butel...
4.3 out of 5
Language | : | English |
File size | : | 15481 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 848 pages |
X-Ray for textbooks | : | Enabled |